GitHub Actions Checkout: Are you looking to streamline your CI/CD pipeline with GitHub Actions? The actions/checkout
action is your gateway to powerful automation. In this comprehensive guide, we’ll explore how this essential action works, its best practices, and real-world examples that will level up your workflow game.
What is GitHub Actions Checkout?
The actions/checkout
action is like your repository’s virtual assistant. It fetches your repository content into the workflow’s workspace, making your code available for subsequent steps in your GitHub Actions workflow. Think of it as preparing your workbench before starting a project.
Why is Checkout Important?
Before diving into the technical details, let’s understand why the checkout action is crucial:
- It’s the foundation for most GitHub Actions workflows
- Enables access to your repository’s code and files
- Allows for testing, building, and deploying your applications
- Supports advanced git operations within your workflows
Basic Usage: Getting Started
The simplest way to use the checkout action is straightforward. Here’s a basic example:
steps:
- uses: actions/checkout@v4
This single line does a lot behind the scenes:
- Clones your repository
- Fetches only the latest commit (for efficiency)
- Sets up the workspace for your workflow
Advanced Features and Examples
1. Checking Out Specific Branches
Need to work with a specific branch? Here’s how:
steps:
- uses: actions/checkout@v4
with:
ref: development
2. Fetching Complete Git History
For scenarios requiring full git history (like versioning):
steps:
- uses: actions/checkout@v4
with:
fetch-depth: 0
3. Working with Private Repositories
Here’s a real-world example of checking out private repositories:
name: Build with Dependencies
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- uses: actions/checkout@v4
with:
repository: my-org/private-tools
path: tools
token: ${{ secrets.PAT_TOKEN }}
Best Practices and Pro Tips
1. Version Pinning
Always specify the version of checkout you’re using. While @v4
is recommended, you can be even more specific:
steps:
- uses: actions/checkout@v4.1.1
2. Optimize for Speed
For large repositories, limit the fetch depth when full history isn’t needed:
steps:
- uses: actions/checkout@v4
with:
fetch-depth: 1
3. Submodules Handling
When working with submodules, configure them explicitly:
steps:
- uses: actions/checkout@v4
with:
submodules: recursive
Common Troubleshooting Scenarios
Issue: Authentication Failed
# Solution
steps:
- uses: actions/checkout@v4
with:
token: ${{ secrets.GITHUB_TOKEN }}
Issue: Sparse Checkout
# For large monorepos
steps:
- uses: actions/checkout@v4
with:
sparse-checkout: true
sparse-checkout-cone-mode: true
sparse-checkout-patterns: |
app/
packages/core/
Real-World Implementation Example
Here’s a complete workflow example that demonstrates several checkout features:
name: Production Deploy
on:
push:
branches:
- main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Checkout Main Repository
uses: actions/checkout@v4
with:
fetch-depth: 0
- name: Checkout Dependencies
uses: actions/checkout@v4
with:
repository: company/shared-libs
path: ./libs
token: ${{ secrets.PAT_TOKEN }}
- name: Build and Deploy
run: |
npm ci
npm run build
npm run deploy
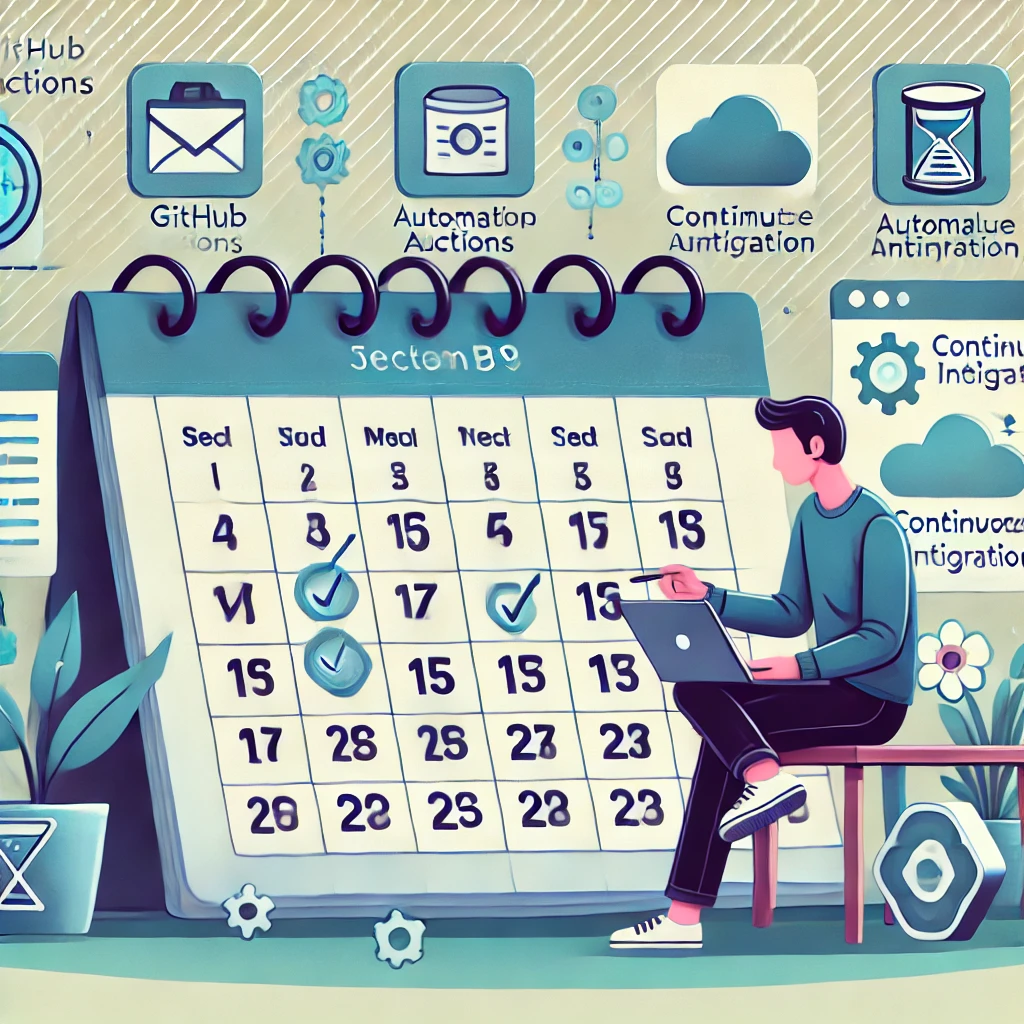
Future-Proofing Your Workflows
The checkout action continues to evolve with new features and improvements. Stay updated by:
- Following the official GitHub Actions changelog
- Regularly reviewing your workflow configurations
- Testing new versions in a development environment before production deployment
Conclusion
The GitHub Actions checkout action is more than just a simple clone operation – it’s a powerful tool that forms the backbone of your CI/CD pipeline. By understanding its features and implementing best practices, you can create more efficient and reliable workflows.
Remember to:
- Always specify versions
- Optimize fetch depth for your needs
- Handle authentication properly
- Consider submodules when necessary
Start implementing these practices today, and watch your GitHub Actions workflows become more robust and maintainable.
Looking to learn more? Check out the official GitHub Actions documentation and join the GitHub Community discussions for more insights and tips.
Recent Post: AWS serverless