As a software engineer who’s implemented authentication for dozens of applications, I can tell you that managing user identity is both crucial and challenging. Enter AWS Cognito – Amazon’s powerful yet often misunderstood authentication service. Let’s dive into everything you need to know about this game-changing tool.
🔐 What is AWS Cognito?
Think of Cognito as your application’s security bouncer – it handles all the complex stuff like user registration, authentication, and access control, so you can focus on building your app’s core features. It’s like having a professional security team that works 24/7, but without the hefty payroll!
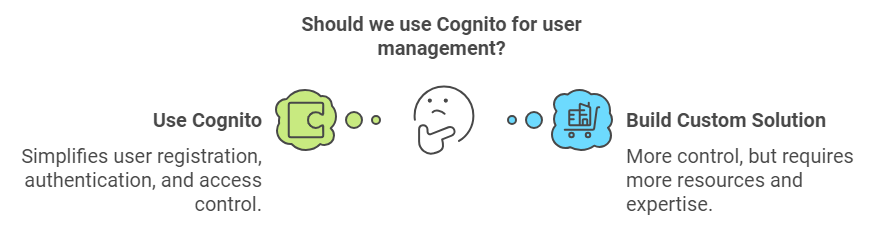
Key Features That Make AWS Cognito Awesome 💪
1. User Pools: Your User Directory on Steroids
User pools are like a turbocharged database for your user accounts. They handle:
- Sign-up and sign-in flows
- Password policies and reset workflows
- Multi-factor authentication (MFA)
- Email and phone verification
2. Identity Pools: Temporary AWS Access
Identity pools (also called Federated Identities) are your ticket to giving users temporary access to other AWS services. Imagine letting users directly upload files to S3 – securely!
3. Social Identity Federation
Want users to log in with their Facebook, Google, or Apple accounts? Cognito’s got you covered. It’s like having a universal translator for different authentication systems.
Real-World Example of AWS Cognito: Building a Secure Photo Sharing App🚀
Let’s walk through a practical example. Imagine we’re building “SnapShare” – a photo-sharing application.
// Initialize the Amazon Cognito credentials provider
const poolData = {
UserPoolId: 'us-east-1_xxxxx',
ClientId: 'abcdef123456'
};
const userPool = new AmazonCognitoIdentity.CognitoUserPool(poolData);
// Sign up a new user
const signUp = async (email, password) => {
try {
const result = await userPool.signUp(email, password, [], null);
console.log('User signed up successfully:', result.user);
} catch (error) {
console.error('Error signing up:', error);
}
};
🛠️ Best Practices I’ve Learned the Hard Way
- Always Enable MFA: It’s like wearing a seatbelt – you hope you never need it, but you’ll be glad it’s there.
- Use Custom Authentication Challenges: Perfect for adding CAPTCHA or step-up authentication.
- Implement Proper Error Handling: Your users will thank you when things go wrong (and they will).
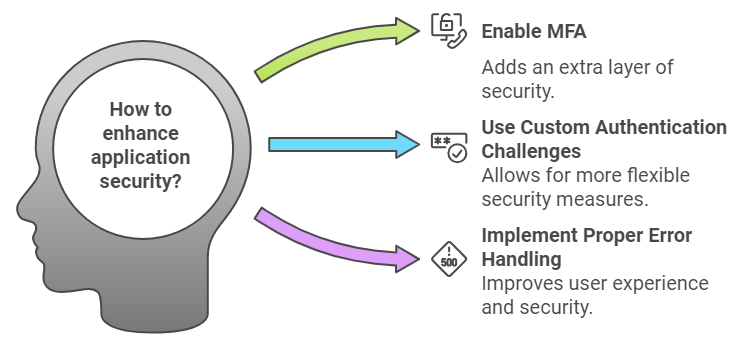
🌟 Pro Tips of AWS Cognito for Advanced Users
- Use Lambda Triggers to customize authentication flows
- Implement Custom Authentication Flow for unique requirements
- Set up User Migration for seamless transitions from legacy systems
⚡ Performance Optimization
Remember to:
- Cache tokens appropriately
- Implement refresh token rotation
- Use connection pooling for high-traffic applications
❓ Frequently Asked Questions
How much does AWS Cognito cost?
Cognito follows a pay-as-you-go model. The first 50,000 monthly active users are free. After that, you pay around $0.0055 per monthly active user. Check out the official pricing page for details.
Can I use Cognito with my existing user database?
Absolutely! Use the User Migration Lambda trigger to seamlessly migrate users during their first login attempt.
Is Cognito HIPAA compliant?
Yes, AWS Cognito is HIPAA eligible and can be used within a HIPAA compliant environment when properly configured.
How does Cognito handle password reset?
Cognito provides built-in forgot password flows with customizable email templates and verification codes.
🎯 Conclusion
AWS Cognito is like having a Swiss Army knife for authentication – it’s versatile, reliable, and gets the job done. While it has its learning curves (trust me, I’ve been there), the benefits far outweigh the initial setup complexity.
Want to dive deeper? Check out these resources:
Remember: good authentication is like good health – you don’t appreciate it until something goes wrong. So invest the time to set up Cognito correctly, and your future self will thank you!
Have you implemented AWS Cognito in your projects? I’d love to hear about your experiences in the comments below!
Next: AWS CloudFront: The Ultimate Guide to Supercharging Your Web Performance 🚀