Hey there, fellow developers! After spending over a decade wrestling with distributed systems, I’ve seen the good, the bad, and the ugly of distributed transactions. Today, I’m breaking down this complex topic into bite-sized pieces. Let’s dive in!
What Are Distributed Transactions? 🤔
Imagine ordering a pizza online. Your payment needs to be processed, the order sent to the restaurant, a delivery driver assigned, and your loyalty points updated. Each of these operations might run on different services, but they all need to succeed (or fail) together. That’s a distributed transaction in action!
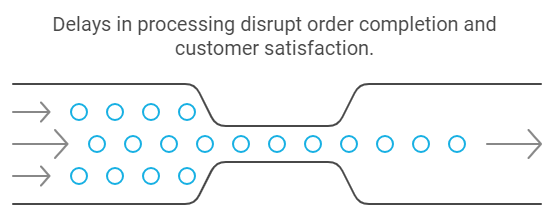
Types of Distributed Transactions
1. Two-Phase Commit (2PC) 🔒
The granddaddy of distributed transactions! Think of 2PC as a wedding ceremony:
- Phase 1 (Prepare): The coordinator (priest) asks all participants (bride, groom) if they’re ready to commit
- Phase 2 (Commit): If everyone says “yes,” the coordinator finalizes the transaction
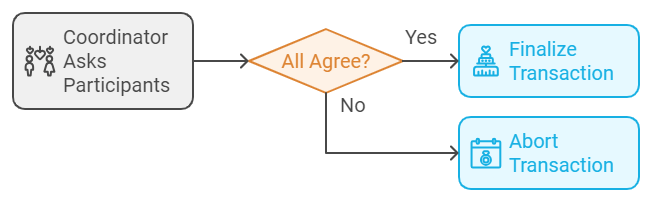
// Pseudo-code example of 2PC
try {
// Phase 1: Prepare
boolean allPrepared = coordinator.prepareAll(participants);
if (allPrepared) {
// Phase 2: Commit
coordinator.commitAll(participants);
} else {
coordinator.rollbackAll(participants);
}
} catch (Exception e) {
coordinator.rollbackAll(participants);
}
Pros:
- Strong consistency
- ACID guarantees
Cons:
- Blocking protocol
- Performance overhead
- Susceptible to coordinator failures
2. Saga Pattern 🔄
My personal favorite and widely used in modern microservices! Instead of one big transaction, we break it down into smaller local transactions, each with a compensation action.
Example using an e-commerce flow:
- Create Order
- Process Payment
- Update Inventory
- Send Notification
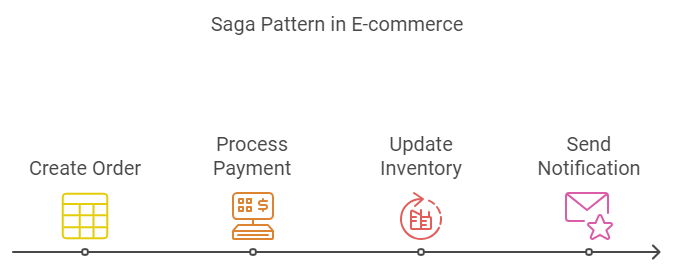
# Pseudo-code for Saga pattern
def create_order_saga():
try:
order = create_order()
payment = process_payment()
inventory = update_inventory()
send_notification()
except PaymentFailure:
compensate_order()
except InventoryFailure:
compensate_payment()
compensate_order()
For a deep dive into Saga implementation, check out Microsoft’s guide on Saga pattern.
3. Eventually Consistent Transactions ⏳
Perfect for scenarios where immediate consistency isn’t critical. Think of social media likes – it’s okay if the count takes a few seconds to update everywhere.
Most Used Distributed Transactions in Practice 🏆
From my experience, the Saga pattern has emerged as the go-to choice for modern distributed systems. Here’s why:
- Non-blocking
- Better scalability
- Natural fit for microservices
- Easier to understand and implement
- Excellent tooling support
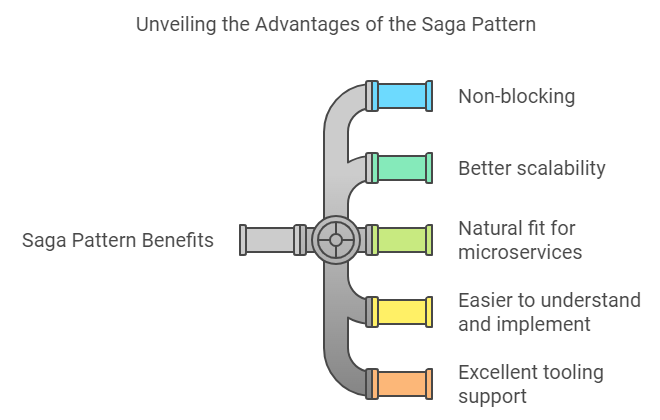
Best Practices 📝
- Always design for failure
- Implement idempotency
- Use event sourcing when possible
- Monitor transaction states
- Implement proper logging
FAQ Section
What is the CAP theorem and how does it relate to distributed transactions? 🤓
The CAP theorem states that a distributed system can only guarantee two out of three properties: Consistency, Availability, and Partition tolerance. In practice, when network partitions occur, you must choose between consistency (2PC) and availability (Saga).
Are distributed transactions always necessary? 🤔
No! Sometimes, you can redesign your system to avoid distributed transactions altogether. For example, you might be able to combine related operations into a single service or use eventual consistency.
How do I handle partial failures in distributed transactions? 💥
Implement compensation logic for each step, use event sourcing to track state changes, and maintain a transaction log for recovery. Tools like Apache Camel can help manage this complexity.
What’s the performance impact of distributed transactions? ⚡
It varies by pattern. 2PC can add significant latency due to its blocking nature. Saga pattern generally performs better but requires more complex error handling. Always benchmark in your specific use case!
Conclusion
Distributed transactions are like conducting an orchestra – every section needs to play its part perfectly to create beautiful music. While they add complexity to our systems, patterns like Saga make them manageable in modern architectures.
Remember, there’s no one-size-fits-all solution. Choose the pattern that best fits your specific requirements, considering factors like consistency needs, scale, and team expertise.
Happy coding! 🚀
Want to dive deeper? Check out my other articles on microservices patterns and distributed systems design.
Next: Unlock Event-Driven Architecture: The Ultimate Guide to AWS EventBridge
2 thoughts on “The Ultimate Guide to Distributed Transactions in 2024”