Hey there, fellow code wranglers! After spending over a decade wrestling with JavaScript modules, I’ve got some insights that’ll save you hours of head-scratching. Let’s break down the epic battle between require
vs import
โ two module loading techniques that have shaped modern JavaScript development.
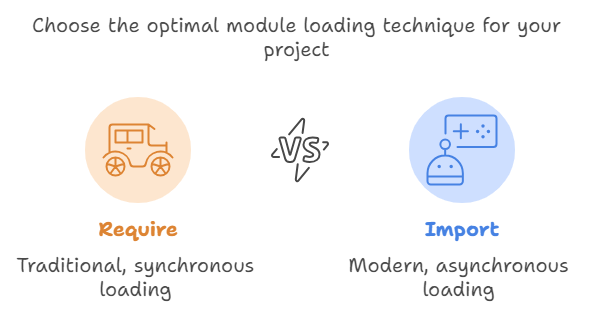
Why Module Systems Matter ๐งฉ
Back in the wild west days of JavaScript, managing code dependencies was like herding cats. Developers struggled to organize and import code efficiently. Then came module systems โ our digital superheroes!
The Evolution of JavaScript Modules
- Pre-ES6: Chaotic module management
- CommonJS (
require
): Node.js breakthrough - ES6 Modules (
import
): Modern JavaScript standard
Require: The OG Module Loader ๐ฐ๏ธ
// Classic CommonJS style
const express = require('express');
const { readFile } = require('fs');
require
is the veteran of module loading, born in the Node.js ecosystem. It’s synchronous, which means it loads modules immediately and blocks execution until the module is fully loaded.
Pros of Require
- Simple, straightforward syntax
- Works seamlessly in Node.js
- Widespread legacy support
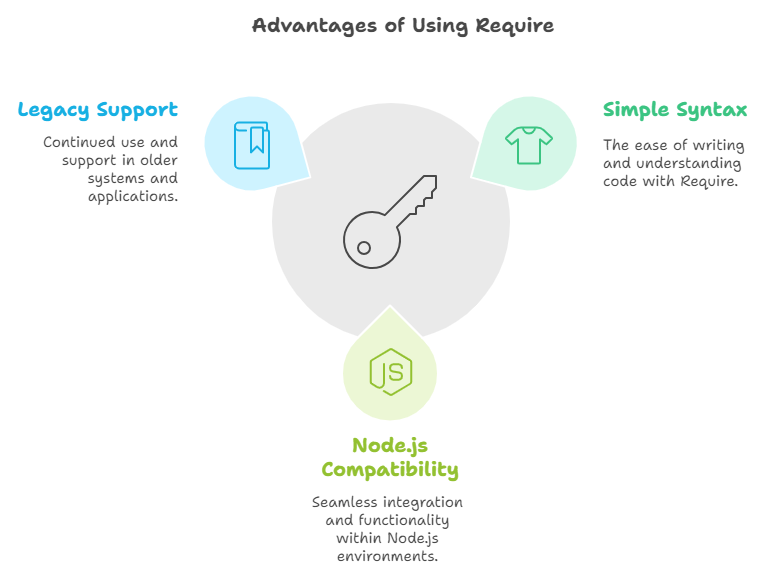
Cons of Require
- Synchronous loading (potential performance hit)
- Limited tree-shaking capabilities
- Less flexible compared to modern imports
Import: The Modern Module Marvel ๐
// ES6 Module magic
import express from 'express';
import { readFile } from 'fs/promises';
import
is the cool new kid on the block, bringing asynchronous loading and more granular control over module imports.
Superpowers of Import
- Asynchronous loading
- Tree-shaking support
- More precise module selection
- Better performance in browser environments
Comparative Breakdown ๐
Feature | require | import |
---|---|---|
Module System | CommonJS | ES6 Modules |
Loading Type | Synchronous | Asynchronous |
Tree Shaking | โ Limited | โ Supported |
Browser Support | โ Native | โ Modern Browsers |
Dynamic Imports | Challenging | Native Support |
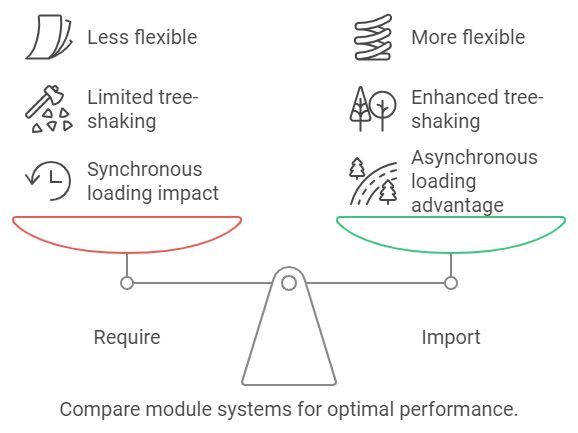
Real-World Scenario Showdown ๐ฅ
Scenario 1: Performance-Critical Web App
// Require (Less Optimal)
const _ = require('lodash');
_.map([1, 2, 3], x => x * 2);
// Import (Performance Winner)
import { map } from 'lodash-es';
map([1, 2, 3], x => x * 2);
The import
version allows for better tree-shaking and smaller bundle sizes.
Scenario 2: Node.js Backend
// Modern Node.js with ES Modules
import express from 'express';
import { connectDB } from './database.js';
const app = express();
await connectDB();
When to Use What? ๐ค
- Use
require
when: - Working with legacy Node.js projects
- Using older libraries without ES Module support
- In pure server-side environments
- Use
import
when: - Building modern web applications
- Working with frontend frameworks
- Prioritizing performance and code splitting
FAQ Section ๐คจ
What exactly is tree-shaking?
Tree-shaking is a dead code elimination technique that removes unused code from your final bundle, dramatically reducing file size.
Can I use import
in Node.js?
Absolutely! Since Node.js 12+, ES Modules are fully supported. You’ll need to use .mjs
extensions or configure package.json
.
How do I convert from require
to import
?
- Use
.mjs
file extensions - Add
"type": "module"
inpackage.json
- Gradually migrate your codebase
Are there performance differences?
import
generally offers better performance due to asynchronous loading and optimized bundling.
Pro Tips ๐ก
- Use tools like Webpack or Rollup for advanced module handling
- Always consider your project’s specific requirements
- Keep an eye on browser and runtime support
Conclusion ๐
Both require
and import
have their place in the JavaScript ecosystem. While import
is clearly the future, understanding both gives you flexibility in diverse project environments.
Want to dive deeper? Check out these resources:
Happy coding, and may your modules always be clean and your bundles light! ๐๐ฉโ๐ป๐จโ๐ป
Next: How B-Tree Indexes Power Lightning-Fast Database Queries ๐