Hey there, fellow developers! After spending over a decade architecting e-commerce systems, I’ve learned that efficient order fulfillment can make or break your business. Today, I’m excited to share how you can build a smart system that automatically fulfills orders from the nearest warehouse using MongoDB and Node.js.
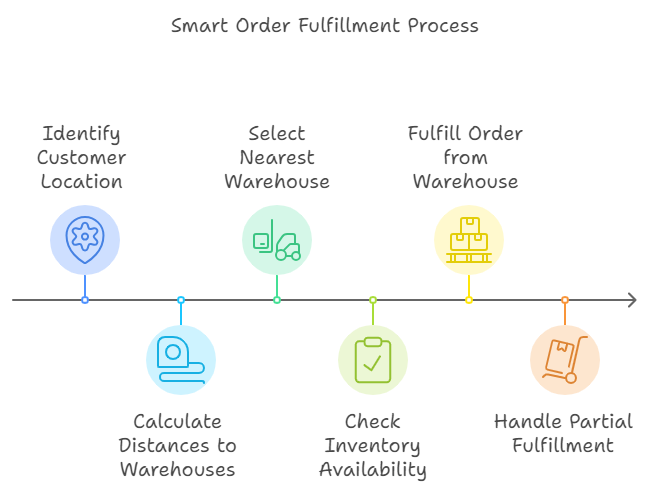
TL;DR: We’ll create a system that finds the closest warehouse to your customer, checks inventory availability, and handles partial fulfillment when needed. All powered by MongoDB’s flexibility and Node.js’s async capabilities.
π¦ Why Build a Smart Warehouse System?
Picture this: Your e-commerce business is booming (congrats! π), but you’re struggling to efficiently fulfill orders from multiple warehouses. Your customers are getting frustrated with delayed deliveries, and your operations team is drowning in manual work. Sound familiar?
Here’s what our system will solve:
- β‘ Automatically find the nearest warehouse to each customer
- π Check real-time inventory across locations
- π Handle partial fulfillment from multiple warehouses
- π° Reduce shipping costs and delivery times
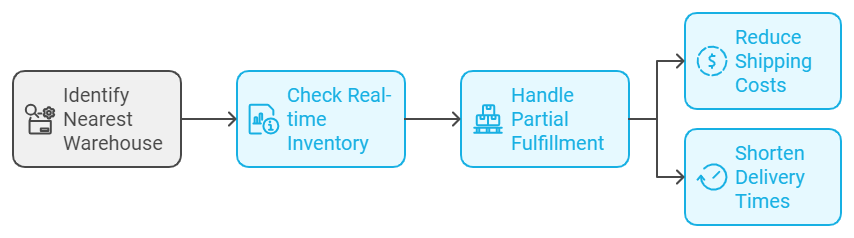
ποΈ Setting Up Our Database Structure
First things first – let’s design our MongoDB schema. We’ll need two main collections:
The Warehouse Collection π
{
"_id": ObjectId("..."),
"name": "Brooklyn Warehouse",
"location": {
"lat": 40.7128,
"long": -74.0060
},
"inventory": [
{ "itemId": "gaming-laptop", "quantity": 100 },
{ "itemId": "wireless-earbuds", "quantity": 200 }
]
}
The Order Collection π
{
"_id": ObjectId("..."),
"customer": "Sarah Johnson",
"items": [
{ "itemId": "gaming-laptop", "quantity": 1 },
{ "itemId": "wireless-earbuds", "quantity": 2 }
],
"location": {
"lat": 40.730610,
"long": -73.935242
},
"status": "pending"
}
π The Magic Behind Distance Calculation
One of the coolest parts of this system is how we calculate distances between locations. We’ll use the Haversine formula, which calculates the shortest distance between two points on a sphere. Pretty neat, right?
function getDistanceFromLatLonInKm(lat1, lon1, lat2, lon2) {
const R = 6371; // Earth's radius in km
const dLat = deg2rad(lat2 - lat1);
const dLon = deg2rad(lon2 - lon1);
const a =
Math.sin(dLat / 2) * Math.sin(dLat / 2) +
Math.cos(deg2rad(lat1)) * Math.cos(deg2rad(lat2)) *
Math.sin(dLon / 2) * Math.sin(dLon / 2);
const c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1 - a));
return R * c; // Distance in km
}
π― Finding the Perfect Warehouse
Now comes the fun part – finding the closest warehouse that can fulfill our order. Here’s our optimized warehouse finder:
const findNearestWarehouse = async (order, db) => {
const warehouses = await db.collection('warehouses').find({}).toArray();
const orderLocation = order.location;
// Map distances and sort warehouses
const sortedWarehouses = warehouses
.map(warehouse => ({
...warehouse,
distance: getDistanceFromLatLonInKm(
orderLocation.lat, orderLocation.long,
warehouse.location.lat, warehouse.location.long
)
}))
.sort((a, b) => a.distance - b.distance);
// Check inventory availability
return sortedWarehouses.find(warehouse =>
order.items.every(item => {
const stock = warehouse.inventory.find(i => i.itemId === item.itemId);
return stock && stock.quantity >= item.quantity;
})
);
};
π Smart Partial Fulfillment
Sometimes, no single warehouse has all the items a customer needs. Instead of canceling the order, our system intelligently splits it across multiple warehouses. Let’s see how this works!
Example Scenario π―
Imagine Sarah orders:
- 3 gaming laptops
- 5 wireless earbuds
But our warehouses have:
// Brooklyn Warehouse
{
"name": "Brooklyn Warehouse",
"inventory": [
{ "itemId": "gaming-laptop", "quantity": 2 },
{ "itemId": "wireless-earbuds", "quantity": 5 }
]
}
// Jersey City Warehouse
{
"name": "Jersey City Warehouse",
"inventory": [
{ "itemId": "gaming-laptop", "quantity": 1 },
{ "itemId": "wireless-earbuds", "quantity": 2 }
]
}
Here’s our smart partial fulfillment logic:
const handlePartialFulfillment = async (order, db) => {
const fulfillmentPlan = [];
// Track remaining quantities for each item
const remainingItems = order.items.map(item => ({
...item,
remainingQuantity: item.quantity
}));
while (remainingItems.some(item => item.remainingQuantity > 0)) {
// Get all warehouses sorted by distance
const warehouses = await findSortedWarehouses(order.location, db);
for (const warehouse of warehouses) {
const warehouseFulfillment = {
warehouseId: warehouse._id,
items: []
};
// Try to fulfill as much as possible from this warehouse
for (const item of remainingItems) {
const inventoryItem = warehouse.inventory.find(i =>
i.itemId === item.itemId && i.quantity > 0
);
if (inventoryItem) {
const fulfillQuantity = Math.min(
item.remainingQuantity,
inventoryItem.quantity
);
if (fulfillQuantity > 0) {
warehouseFulfillment.items.push({
itemId: item.itemId,
quantity: fulfillQuantity
});
// Update remaining quantities
item.remainingQuantity -= fulfillQuantity;
inventoryItem.quantity -= fulfillQuantity;
}
}
}
if (warehouseFulfillment.items.length > 0) {
fulfillmentPlan.push(warehouseFulfillment);
// Update warehouse inventory in database
await updateWarehouseInventory(warehouse, db);
}
}
// Check if we couldn't fulfill anything in this round
if (!fulfillmentPlan.length) {
throw new Error('Unable to fulfill order with current inventory');
}
}
return fulfillmentPlan;
};
How Partial Fulfillment Works π
- Initial Check: The system first tries to find a single warehouse that can fulfill the entire order (as we saw earlier).
- Split Strategy: If no single warehouse can handle it, we:
- Sort warehouses by distance to minimize shipping costs
- Track remaining quantities needed for each item
- Build a fulfillment plan across multiple warehouses
- Smart Allocation: For Sarah’s order:
- Brooklyn Warehouse provides:
- 2 gaming laptops
- 5 wireless earbuds
- Jersey City Warehouse provides:
- 1 gaming laptop
- Inventory Updates: The system atomically updates inventory across warehouses using MongoDB transactions.
Handling Edge Cases β οΈ
Our system also handles several critical scenarios:
const handleEdgeCases = async (fulfillmentPlan, order, db) => {
// Handle insufficient total inventory
if (!canFulfillCompletely(fulfillmentPlan, order)) {
await notifyInventoryTeam(order);
await updateOrderStatus(order, 'partially-fulfilled');
}
// Handle shipping cost optimization
if (fulfillmentPlan.length > 1) {
await calculateConsolidatedShipping(fulfillmentPlan);
}
// Track split shipments
await createShipmentTracking(fulfillmentPlan, order);
};
Pro Tips for Partial Fulfillment π‘
- Always prioritize filling complete sets of items from the same warehouse
- Consider implementing a maximum split limit (e.g., no more than 3 warehouses per order)
- Add weight and shipping cost calculations to optimize the split strategy
- Implement customer communication for split shipments
π Putting It All Together with Express
Let’s wrap everything in a nice Express API:
const express = require('express');
const { MongoClient } = require('mongodb');
const app = express();
app.use(express.json());
MongoClient.connect('mongodb://localhost:27017', async (err, client) => {
const db = client.db('warehouse-system');
app.post('/api/orders', async (req, res) => {
try {
const result = await fulfillOrder(req.body, db);
res.json(result);
} catch (error) {
res.status(500).json({ error: error.message });
}
});
app.listen(3000, () => console.log('Server running! π'));
});
π Performance Optimization Tips
- Use MongoDB’s Geospatial Indexes: Speed up location queries with 2dsphere indexes.
- Implement Caching: Use Redis for frequently accessed inventory data.
- Batch Updates: Group inventory updates to reduce database load.
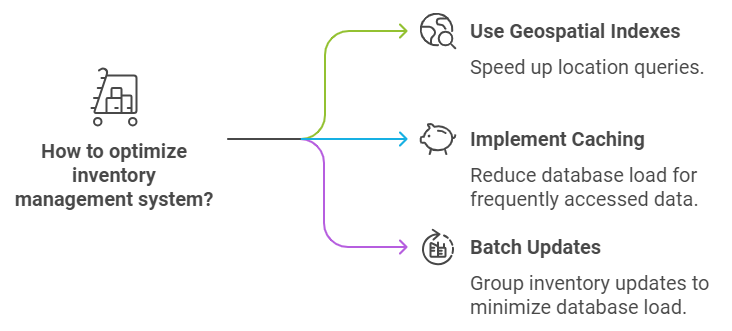
β Frequently Asked Questions
How does the system handle failed deliveries?
The system includes a retry mechanism and status tracking. Failed deliveries trigger automatic re-routing to the next nearest warehouse with available inventory.
What happens if no warehouse has enough inventory?
In this case, the system implements a backorder strategy, notifying customers about expected fulfillment dates based on incoming inventory data.
Can this system scale for enterprise use?
Absolutely! With proper indexing and MongoDB sharding, this system can handle millions of orders. Consider using message queues like RabbitMQ for better load handling.
How can I ensure data consistency across warehouses?
We recommend using MongoDB transactions and implementing an event-driven architecture using something like Apache Kafka for real-time inventory synchronization.
How do you handle partial fulfillment shipping costs?
The system calculates optimal shipping routes and may consolidate items at a central location if it’s more cost-effective than multiple direct shipments.
What happens if an item becomes unavailable during partial fulfillment?
We use MongoDB transactions to lock inventory during the fulfillment process, preventing race conditions. If an item becomes unavailable, the system automatically recalculates the fulfillment plan.
π¬ Wrapping Up
Building a smart warehouse fulfillment system might seem daunting, but with the right tools and architecture, it’s totally achievable. Remember to:
- Start Small: Begin with core functionality and expand
- Monitor Performance: Use tools like MongoDB Compass to track query performance
- Plan for Scale: Design with growth in mind from day one
Happy coding! π
Next: Unlock Event-Driven Architecture: The Ultimate Guide to AWS EventBridge