Overview
Authentication and permission are essential components in protecting sensitive data in the digital world, where security lapses and illegal access persist. Apollo offers a useful framework for developers using GraphQL to expedite these procedures. We’ll examine the fundamentals of Apollo authentication and authorization in this post, dissecting their importance, approaches, and recommended practices.
Describe authorization and authentication.
The First Line of Defense: Authentication
The process of confirming a user’s or system’s identification is called authentication. It guarantees that the individual or organization attempting to use a resource is who they say they are. Typical authentication techniques include:
- Password and Username: The most conventional and popular approach.
- Token-Based Authentication: More secure methods are offered by systems such as JWT (JSON Web Tokens) and OAuth.
- Multi-Factor Authentication (MFA): This method improves security by requiring two or more verification techniques.
Consider authentication as the process by which a club bouncer verifies IDs before admitting patrons.
Permission: Providing Entry
Authorization establishes what resources and actions a user is permitted to take after their identity has been verified. Roles or attributes may serve as the foundation for this (role-based access control or attribute-based access control). A closer look at the various forms of authorization is provided below:
- Role-Based Access Control (RBAC): Access is granted based on a user’s role within an organization, such as admin, editor, or viewer.
- Attribute-Based Access Control (ABAC): Access is granted based on attributes of users, resources, and the environment, allowing for more complex and context-aware permission settings.
Imagine authorization as the staff at the club, which allows patrons to enter specific areas based on their ticket type or VIP status.
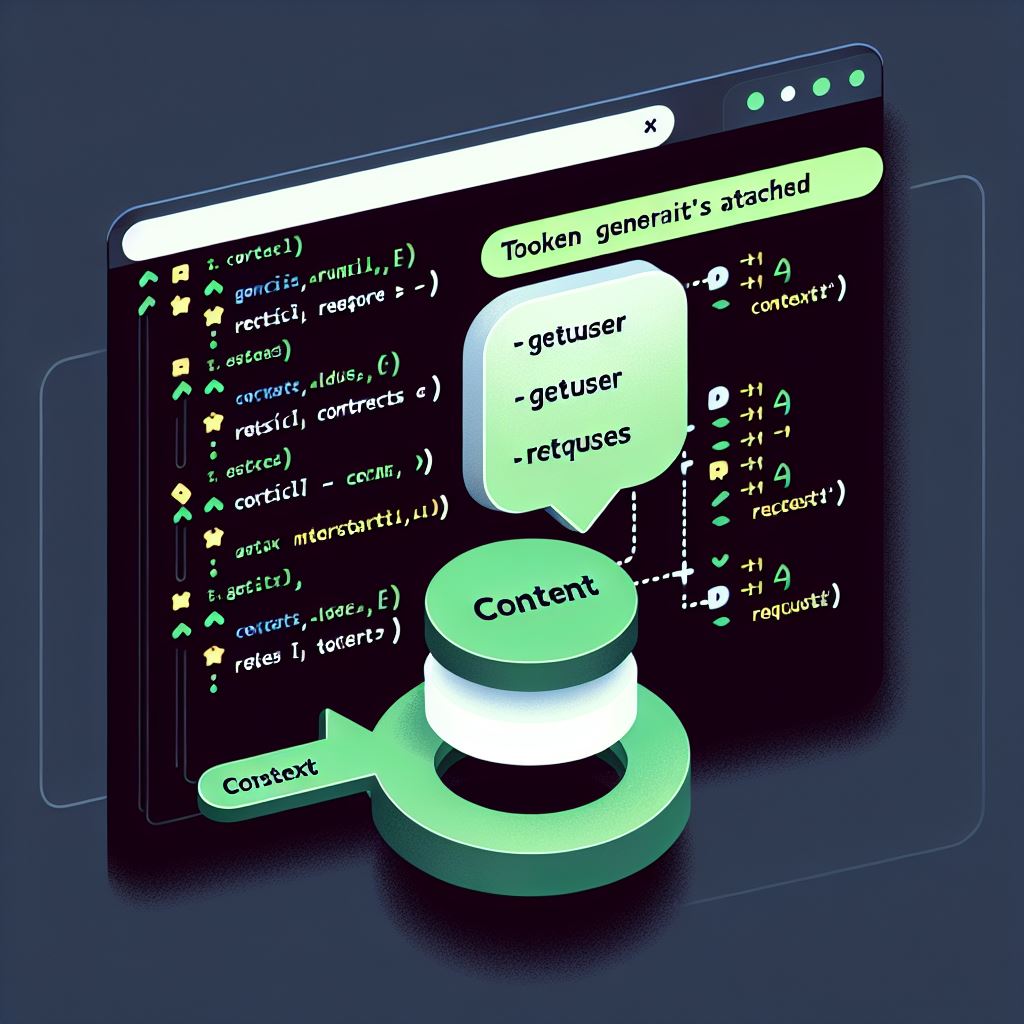
Setting Up Apollo Authentication
Integrating Authentication in Apollo Server
To implement authentication in Apollo Server, follow these steps:
- User Registration: Create a system where users can sign up and provide their credentials.
- Generate Tokens: Upon successful authentication, generate a JWT or another type of token.
- Attach Tokens to Requests: Clients must include these tokens in the headers of requests whenever they access protected routes.
Here’s a basic example of middleware in Apollo Server to demonstrate authentication:
const { AuthenticationError } = require('apollo-server');
const getUser = (token) => {
// Logic to verify token and return user data
}
const context = ({ req }) => {
const token = req.headers.authorization || '';
const user = getUser(token);
if (!user) {
throw new AuthenticationError('You must be logged in');
}
return { user };
};
Best Practices for Implementing Authentication
To ensure robust authentication, consider the following:
- Encrypt Passwords: Use bcrypt or similar libraries to hash passwords before storing them.
- Implement Token Expiration: Set tokens to expire after a certain period and allow for refresh tokens.
- Monitor Failed Login Attempts: Keep track of repeated login failures to detect suspicious activity.
Streamlining Authorization in Apollo
Role-Based Access Control (RBAC)
Implementing RBAC in your Apollo application involves defining roles and managing permissions accordingly. Here’s how you can do it:
- Define Roles: Clearly outline the various roles within your application and their corresponding permissions.
- Middleware for Role Verification: Create middleware that checks if a user has the necessary role before granting access.
Here’s an example of a role-checking middleware:
const checkUserRole = (role) => {
return (next) => (root, args, context, info) => {
if (!context.user || context.user.role !== role) {
throw new ForbiddenError('You do not have access');
}
return next(root, args, context, info);
};
};
// Usage in type definitions
const resolvers = {
Query: {
secretData: checkUserRole('admin')(someSecretDataResolver),
},
};
Access Control Based on Attributes (ABAC)
ABAC offers a more granular method that enables dynamic authorization according to several characteristics. To implement ABAC, you must:
- Defining Attributes: List the different attributes, including user department and project status, that can affect access control.
- Policies: Establish policies that compare these properties to the context of the request.
Advice for Effective Permission
To facilitate the authorization process for you:
- Keep It Simple: Systems that are too complicated may be vulnerable. Make use of precise, unambiguous permissions criteria.
- Regular Audits: Verify that user responsibilities and permissions are in line with the existing organizational structure on a regular basis.
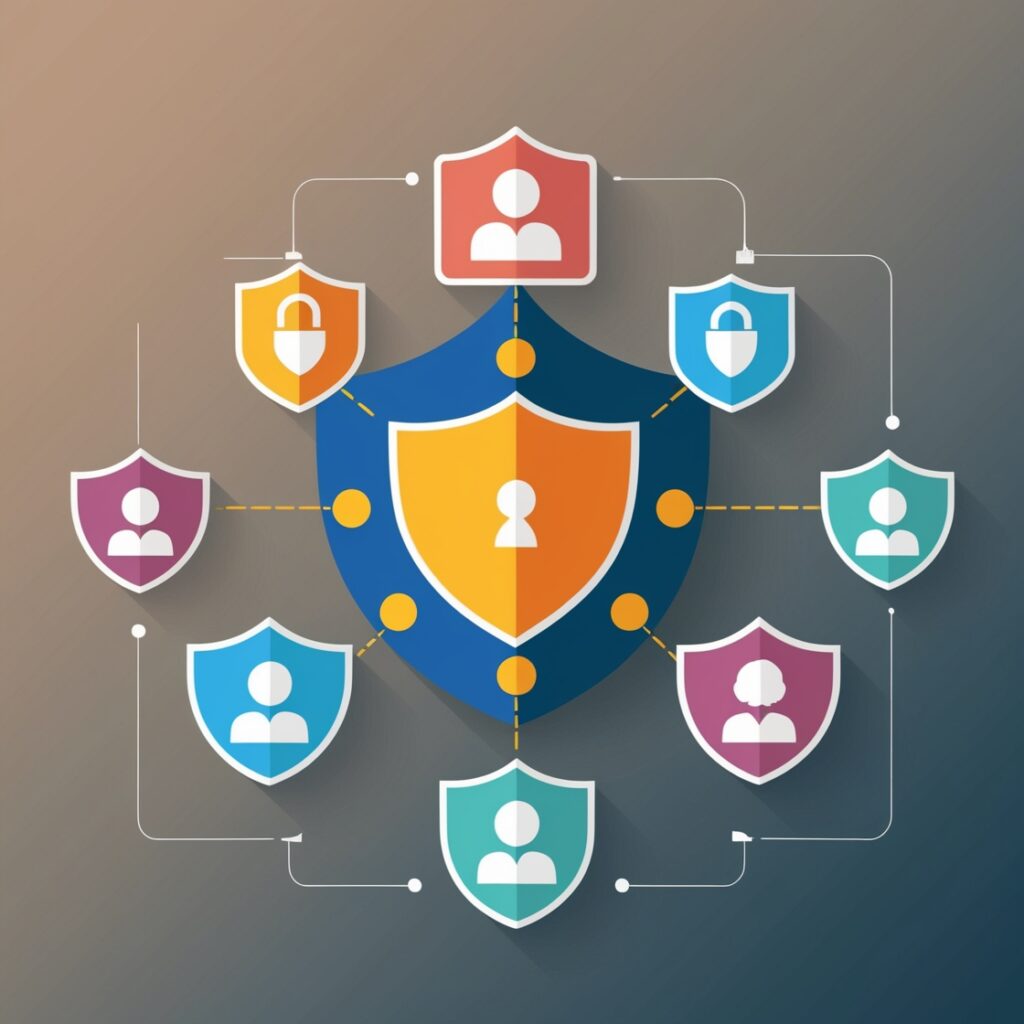
Wrap-up
Authorization and authentication are essential components in preserving the security and integrity of Apollo applications. By putting these procedures into practice well, you may improve user engagement and confidence while simultaneously protecting sensitive data.
To provide a secure environment for your users, think about using strong authentication and authorization techniques when you develop your applications. Resources like the official Apollo Server documentation can be very helpful for developers who are new to Apollo.
“Security is not a product, but a process.” Bruce Schneier
Spend some time investigating permission and authentication alternatives further, adjusting them to the particular requirements of your project. Have you added authorization and authentication to your apps? What difficulties did you encounter and how did you resolve them? Leave a comment with your experiences.
1 thought on “A Complete Guide to Apollo Authentication and Authorization Understanding”