Are you building a Node.js application and wondering how to keep your users’ data safe? You’re in the right place! Today, we’ll dive into Node.js’s built-in nodejs crypto
module – your Swiss Army knife for all things cryptography. Whether you’re handling passwords, securing API endpoints, or managing sensitive data, this guide has got you covered.
What is the Nodejs Crypto Module?
The crypto
module is Node.js’s powerful built-in tool that helps you implement cryptographic functionality. Think of it as your security toolkit – it’s like having a professional locksmith’s set of tools right in your code!
🔑 Common Use Cases of Nodejs crypto
1. Hashing Passwords
Let’s start with something you’ll use almost every day – hashing passwords. Here’s a simple example:
const crypto = require('crypto');
function hashPassword(password) {
// Create a random salt
const salt = crypto.randomBytes(16).toString('hex');
// Hash the password with the salt
const hash = crypto.pbkdf2Sync(password, salt, 1000, 64, 'sha512').toString('hex');
return { salt, hash };
}
// Usage example
const userPassword = 'mySecurePassword123';
const { salt, hash } = hashPassword(userPassword);
console.log(`Salt: ${salt}\nHash: ${hash}`);
2. Generating Random Tokens
Need to create secure reset password tokens or session IDs? Here’s how:
function generateSecureToken(length = 32) {
return crypto.randomBytes(length).toString('hex');
}
// Create a secure reset password token
const resetToken = generateSecureToken();
console.log(`Reset Token: ${resetToken}`);
3. Encrypting and Decrypting Data
Sometimes you need to store sensitive data that you’ll need to retrieve later. Here’s a practical example:
function encryptData(data, secretKey) {
const iv = crypto.randomBytes(16);
const cipher = crypto.createCipheriv('aes-256-gcm', secretKey, iv);
let encrypted = cipher.update(data, 'utf8', 'hex');
encrypted += cipher.final('hex');
const authTag = cipher.getAuthTag();
return {
iv: iv.toString('hex'),
encrypted,
authTag: authTag.toString('hex')
};
}
function decryptData(encryptedData, secretKey) {
const decipher = crypto.createDecipheriv(
'aes-256-gcm',
secretKey,
Buffer.from(encryptedData.iv, 'hex')
);
decipher.setAuthTag(Buffer.from(encryptedData.authTag, 'hex'));
let decrypted = decipher.update(encryptedData.encrypted, 'hex', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
}
// Usage example
const secretKey = crypto.randomBytes(32);
const sensitiveData = "My secret message";
const encrypted = encryptData(sensitiveData, secretKey);
console.log('Encrypted:', encrypted);
const decrypted = decryptData(encrypted, secretKey);
console.log('Decrypted:', decrypted);
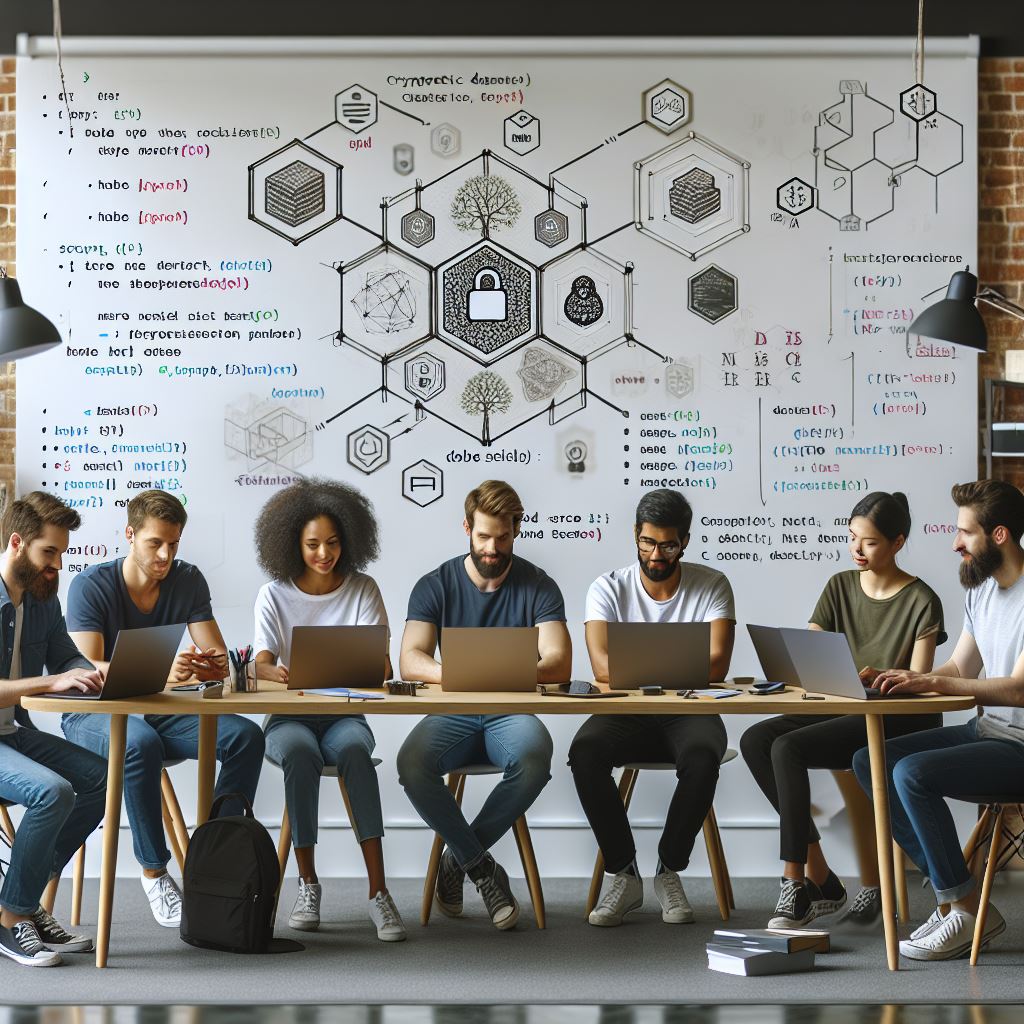
📊 Best Practices
- Never Store Plain Passwords: Always hash passwords using strong algorithms like bcrypt or Argon2.
- Use Secure Random Numbers: Always use
crypto.randomBytes()
instead ofMath.random()
for security-critical operations. - Keep Your Keys Safe: Store encryption keys securely using environment variables or secure key management systems.
- Update Regularly: Keep your Node.js version updated to get the latest security improvements.
🚀 Performance Considerations
When working with crypto operations, remember that they’re CPU-intensive. Here are some tips:
- Use async versions of crypto functions for better performance
- Consider using a worker thread pool for heavy crypto operations
- Cache results when possible (but be careful with security implications)
🛡️ Real-world Implementation Example
Here’s a practical example of implementing secure user authentication:
const crypto = require('crypto');
class UserAuth {
constructor() {
this.users = new Map();
}
registerUser(username, password) {
const { salt, hash } = this.hashPassword(password);
this.users.set(username, { salt, hash });
return true;
}
verifyUser(username, password) {
const user = this.users.get(username);
if (!user) return false;
const hashVerify = crypto.pbkdf2Sync(
password,
user.salt,
1000,
64,
'sha512'
).toString('hex');
return hashVerify === user.hash;
}
hashPassword(password) {
const salt = crypto.randomBytes(16).toString('hex');
const hash = crypto.pbkdf2Sync(
password,
salt,
1000,
64,
'sha512'
).toString('hex');
return { salt, hash };
}
}
// Usage example
const auth = new UserAuth();
auth.registerUser('john_doe', 'secure123');
console.log(auth.verifyUser('john_doe', 'secure123')); // true
console.log(auth.verifyUser('john_doe', 'wrong_password')); // false
🎯 Common Pitfalls to Avoid
- Using deprecated crypto methods
- Implementing your own encryption algorithms
- Using weak keys or predictable IVs
- Not handling errors properly
🔮 What’s Next?
The crypto landscape is always evolving. Keep an eye on:
- Web Crypto API integration
- Post-quantum cryptography
- New encryption standards
Conclusion
Security is not a feature – it’s a necessity. The Node.js crypto module gives you the tools you need to implement robust security in your applications. Start small, follow best practices, and gradually build up your security infrastructure.
Remember: the best security is the one that’s properly implemented and regularly updated. Happy coding, and stay secure! 🚀
Have questions or want to share your experience with Node.js crypto? Drop a comment below!
Next: Boost Your Coding Efficiency with Cursor AI: The Ultimate AI-Powered Coding Assistant