Ever stuck a password directly in your code and thought, “I’ll fix this later”? Don’t worry – we’ve all been there! But in today’s world of increasing security threats, properly managing your application secrets isn’t just good practice – it’s essential. Let’s dive into AWS Secrets Manager, your new best friend for handling sensitive information.
What is AWS Secrets Manager? 🤔
Think of AWS Secrets Manager as your ultra-secure digital vault. It’s like having a personal security guard for all your sensitive information – database credentials, API keys, OAuth tokens, and other secrets your applications need to function.
Key Features That Make It Awesome ⭐
- Automatic Rotation: Automatically updates your secrets on a schedule
- Fine-grained Access Control: Uses IAM policies to control who can access what
- Encryption: Uses AWS KMS for top-notch encryption
- Cross-Region Replication: Keep your secrets synchronized across different regions
- Centralized Management: One place to rule all your secrets
Real-World Examples: Let’s Get Our Hands Dirty 💻
Example 1: Storing Database Credentials
Here’s how you might store and retrieve database credentials:
import boto3
import json
def get_secret():
session = boto3.session.Session()
client = session.client(
service_name='secretsmanager',
region_name='us-west-2'
)
try:
secret_value = client.get_secret_value(
SecretId='prod/MyApp/PostgreSQL'
)
return json.loads(secret_value['SecretString'])
except Exception as e:
print(f"Error: {str(e)}")
raise e
# Using the secret
credentials = get_secret()
db_username = credentials['username']
db_password = credentials['password']
Example 2: Rotating API Keys 🔄
Let’s say you’re using a third-party payment service. Here’s how you might set up automatic rotation:
- Create a secret with version staging labels:
aws secretsmanager create-secret \
--name prod/MyApp/PaymentAPI \
--secret-string '{"apiKey": "current-key", "apiSecret": "current-secret"}' \
--description "Payment service API credentials"
- Enable automatic rotation:
aws secretsmanager rotate-secret \
--secret-id prod/MyApp/PaymentAPI \
--rotation-lambda-arn arn:aws:lambda:region:account:function:MyRotationFunction \
--rotation-rules '{"AutomaticallyAfterDays": 30}'
Best Practices for Success 📝
- Use Resource Tags 🏷️
- Organize secrets by environment (dev/staging/prod)
- Track cost allocation
- Manage access control
- Implement Least Privilege 👮♂️
- Only give applications access to secrets they need
- Use separate secrets for different environments
- Monitor Access 📊
- Enable CloudTrail logging
- Set up alerts for unauthorized access attempts
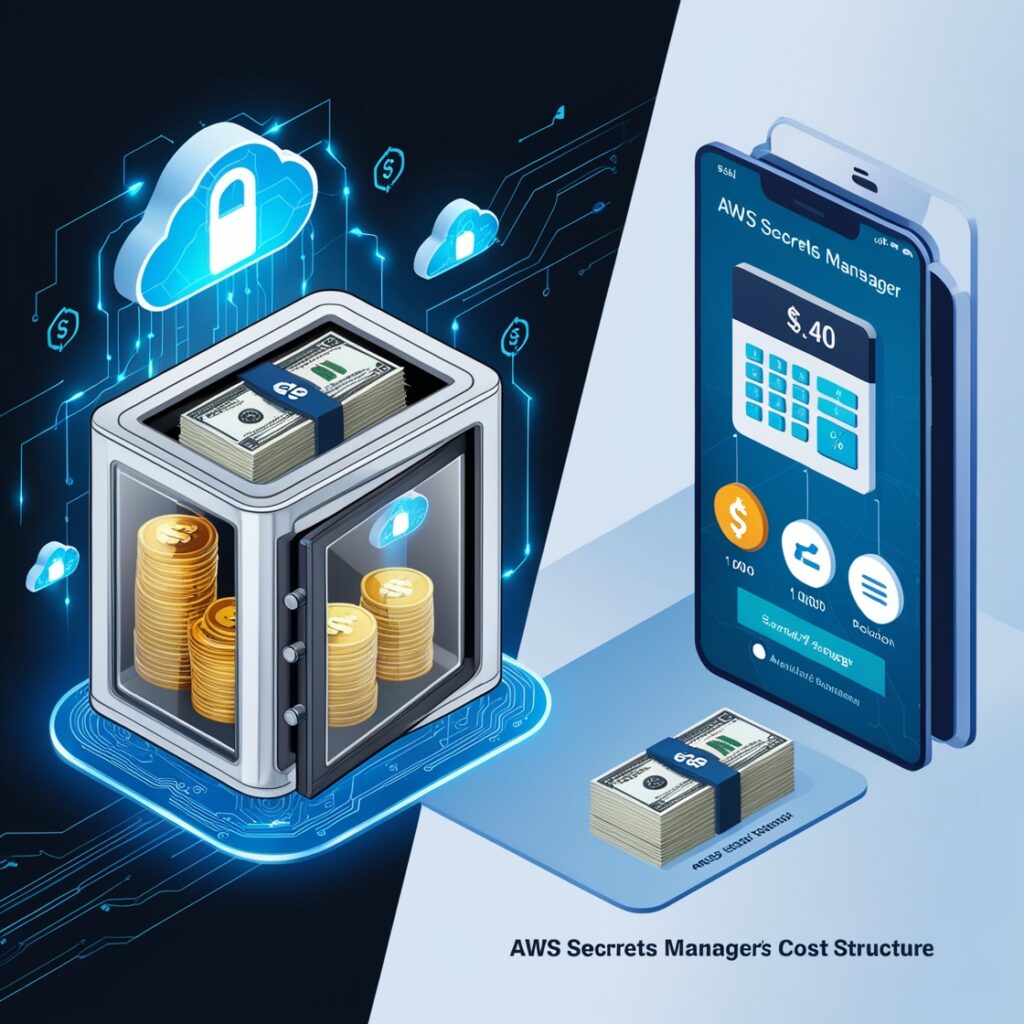
AWS Secrets Manager Cost Considerations 💰
AWS Secrets Manager isn’t free, but it’s probably cheaper than a security breach! Here’s what you’re looking at:
- $0.40 per secret per month
- $0.05 per 10,000 API calls
- No additional charge for automatic rotation
Common Gotchas and How to Avoid Them ⚠️
- Secret Size Limits
- Maximum size: 64KB
- Solution: Split large secrets into multiple smaller ones
- API Rate Limits
- Default: 1000 requests per second
- Solution: Implement caching in your application
- Cross-Account Access
- Can be tricky to set up
- Solution: Use resource-based policies
Getting Started in 5 Minutes ⚡
- Create Your First Secret
aws secretsmanager create-secret \
--name my-first-secret \
--secret-string '{"username":"admin","password":"my-secure-password"}'
- Retrieve It in Your Application
secret = client.get_secret_value(SecretId='my-first-secret')
- Start Sleeping Better at Night 😴
Conclusion 🎯
AWS Secrets Manager isn’t just another tool – it’s your partner in keeping your application’s sensitive data secure. While it might take a bit of time to set up initially, the peace of mind and security it provides are well worth the effort.
Remember: The best time to start properly managing your secrets was when you first wrote your application. The second best time is now!
Ready to Level Up Your Security Game? 🚀
Start with one secret, then gradually migrate your sensitive data. Before you know it, you’ll wonder how you ever managed without it!
Got questions about AWS Secrets Manager? Drop them in the comments below, and let’s learn together!
Next: Mastering AWS AppSync: The Ultimate Guide to Crafting Scalable and Dynamic GraphQL APIs