Introduction
Hey there, fellow tech enthusiasts! After spending over a decade building and optimizing database systems, I’m excited to share my insights about one of the most fascinating data structures in modern computing – the Log-Structured Merge Tree (LSM Tree).
What is an LSM Tree? 🤔
An LSM Tree is a data structure designed to handle high-volume write operations efficiently while maintaining good read performance. Think of it as a clever filing system that’s particularly good at handling tons of new documents quickly.
Visual Representation
Memory (C0):
[Recent Data] ⚡️
↓
Disk (C1):
[Sorted Data] 📝
↓
Disk (C2):
[More Sorted Data] 📚
How Does it Work? ⚙️
- MemTable Stage 🎯
- New writes go to an in-memory buffer (MemTable)
- Usually implemented as a balanced tree (like Red-Black Tree)
- Super fast write operations
- SSTable Formation 💾
- When MemTable reaches capacity, it’s flushed to disk
- Creates Sorted String Tables (SSTables)
- Data is sorted for efficient retrieval
Here’s a simple Node.js example of a basic MemTable implementation:
class MemTable {
constructor() {
this.data = new Map();
this.threshold = 1000; // Number of entries before flush
}
put(key, value) {
this.data.set(key, {
value,
timestamp: Date.now()
});
if (this.data.size >= this.threshold) {
this.flush();
}
}
get(key) {
return this.data.get(key)?.value;
}
flush() {
// Sort data and write to SSTable
const sortedEntries = [...this.data.entries()].sort();
// Implementation for writing to disk
console.log('Flushing to disk:', sortedEntries);
this.data.clear();
}
}
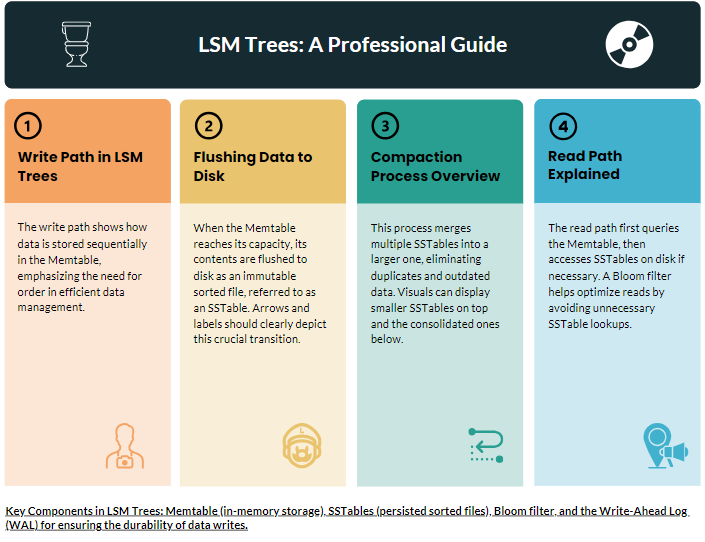
Benefits of LSM Trees 🌟
- High Write Performance ⚡️
- Sequential writes are faster than random writes
- Perfect for write-heavy applications
- Ideal for time-series data
- Space Efficient 📦
- Compaction process removes duplicates
- Better compression ratios
- Reduced disk space usage
- Scalability 🚀
- Handles large datasets efficiently
- Works well with distributed systems
- Used in databases like Cassandra and RocksDB
Real-World Applications 🌍
- Time-Series Databases
- Monitoring systems
- Financial data
- IoT applications
- Key-Value Stores
- Caching systems
- Document databases
- Blockchain implementations
Performance Considerations 📊
Here’s a performance comparison with traditional B-Trees:
Operation | LSM Tree | B-Tree |
---|---|---|
Writes | O(1) | O(log N) |
Reads | O(log N) | O(log N) |
Space | Better | Good |
Best Practices 💡
- Tune Compaction Settings
const compactionOptions = {
level0_filenum_compaction_trigger: 4,
max_bytes_for_level_base: 256 * 1024 * 1024,
write_buffer_size: 64 * 1024 * 1024
};
- Monitor Memory Usage
function checkMemoryUsage() {
const used = process.memoryUsage().heapUsed / 1024 / 1024;
console.log(`Memory usage: ${Math.round(used * 100) / 100} MB`);
}
FAQ Section
What makes LSM Trees different from B-Trees? 🤓
LSM Trees optimize for write performance by using sequential disk I/O, while B-Trees provide more balanced read/write performance with random I/O.
Are LSM Trees good for read-heavy workloads? 📚
While LSM Trees can handle reads well, they’re optimized for writes. For read-heavy workloads, consider using a B-Tree based system or implementing proper caching.
How does compaction work? 🔄
Compaction merges multiple SSTables into larger ones, removing duplicates and organizing data for better read performance. It’s similar to defragmenting a hard drive.
Can LSM Trees handle concurrent operations? 👥
Yes! Modern implementations use techniques like lock-free algorithms and MVCC (Multi-Version Concurrency Control) to handle concurrent operations efficiently.
Useful Resources 📚
Conclusion 🎉
LSM Trees are a powerful data structure that’s revolutionized modern database systems. Their ability to handle high-write workloads while maintaining decent read performance makes them perfect for many modern applications.
Remember: The key to getting the most out of LSM Trees is understanding your workload and tuning accordingly. Happy coding! 🚀
Did you find this article helpful? Don’t forget to share it with your fellow developers and leave a comment below! 💬
Next: How B-Tree Indexes Power Lightning-Fast Database Queries 🚀
1 thought on “The Ultimate Guide to LSM Tree: Boost Database Performance by 10x”