Hey there, fellow code warriors! After spending over a decade wrestling with different programming languages, I’ve developed a special appreciation for TypeScript’s type system. Today, let’s dive deep into one of its most interesting features – Typescript Enum. Trust me, they’re cooler than they sound!
What Are TypeScript Enum? 🤔
Enums (short for enumerations) are a way to define a set of named constants. Think of them as a group of related values that you can use to make your code more readable and maintainable.
enum Direction {
Up = "UP",
Down = "DOWN",
Left = "LEFT",
Right = "RIGHT"
}
Why Should You Care? 💡
- Type Safety: No more magic strings or numbers floating around
- IntelliSense Support: Your IDE becomes your best friend
- Self-Documenting Code: Your future self will thank you
- Reduced Bugs: Catch errors at compile-time instead of runtime
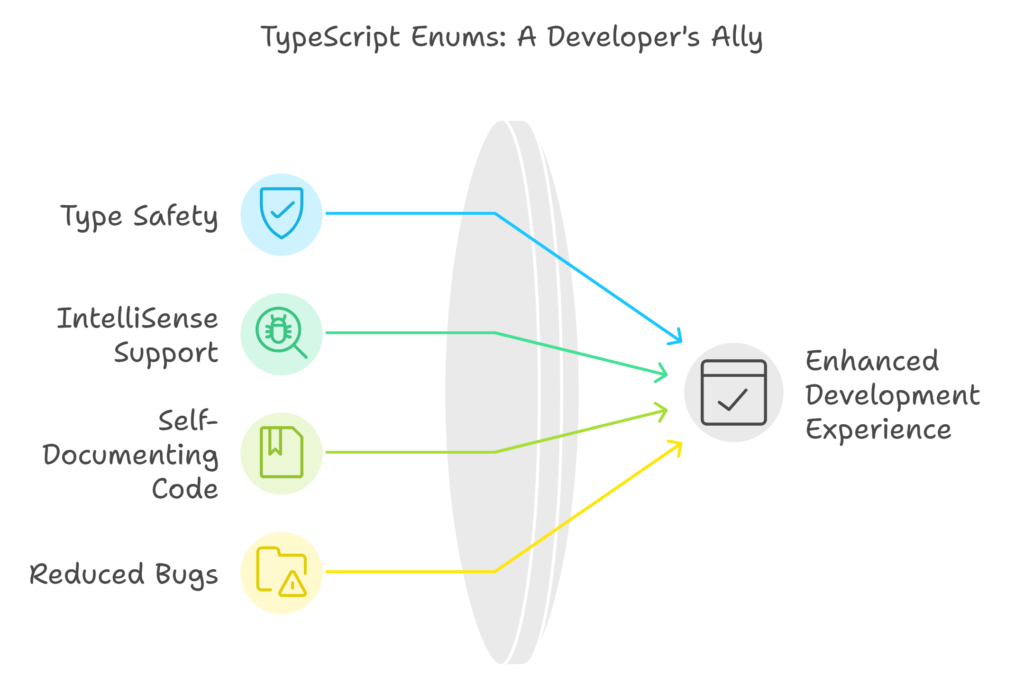
Real-World Examples 🌟
Example 1: User Roles
Let’s say you’re building an authentication system:
enum UserRole {
Admin = "ADMIN",
Editor = "EDITOR",
Viewer = "VIEWER"
}
interface User {
name: string;
role: UserRole;
}
function hasAdminAccess(user: User): boolean {
return user.role === UserRole.Admin;
}
Example 2: API Status Codes
Here’s how you might handle API responses:
enum APIStatus {
Success = 200,
BadRequest = 400,
Unauthorized = 401,
NotFound = 404,
ServerError = 500
}
function handleResponse(statusCode: APIStatus) {
switch (statusCode) {
case APIStatus.Success:
return 'Everything went well!';
case APIStatus.NotFound:
return 'Oops, resource not found!';
// ... handle other cases
}
}
Best Practices to use Typescript Enum ✨
- Use Const Enums for better performance:
const enum Performance {
High = "HIGH",
Medium = "MEDIUM",
Low = "LOW"
}
- Always Initialize String Enums to make the code more maintainable:
enum Visibility {
Public = "PUBLIC",
Private = "PRIVATE",
Protected = "PROTECTED"
}
- Keep Enums Small and focused on a single concept
Common Gotchas and How to Avoid Them ⚠️
- Numeric Enum Auto-Increment
enum Wrong {
A, // 0
B, // 1
C // 2
}
// Better approach
enum Better {
A = 1,
B = 2,
C = 3
}
- Reverse Mappings (only available in numeric enums)
enum Numbers {
One = 1,
Two = 2
}
console.log(Numbers[1]); // "One"
Integration with Modern JavaScript Ecosystem 🔄
TypeScript enums work great with popular frameworks like React and Angular. They’re especially useful when working with state management libraries like Redux.
enum ActionTypes {
FetchData = 'FETCH_DATA',
UpdateUser = 'UPDATE_USER',
DeleteItem = 'DELETE_ITEM'
}
// In your Redux action creators
const fetchData = () => ({
type: ActionTypes.FetchData
});
FAQs 🤓
What’s the difference between const enum and regular enum?
Const enums are completely removed during compilation and inlined wherever they’re used, resulting in better performance. Regular enums are converted to regular JavaScript objects.
Can I use enums in JavaScript?
Pure JavaScript doesn’t have enums, but you can simulate them using Object.freeze() with regular objects.
Should I use string or numeric enums?
String enums are generally preferred as they provide better debugging experience and are more readable in compiled JavaScript code.
Can enums be used in switch statements?
Yes! Enums work perfectly with switch statements and TypeScript will even warn you about unhandled enum values.
Wrapping Up 🎉
Enums might seem like a small feature, but they’re a powerful tool for writing cleaner, more maintainable TypeScript code. They bring type safety and clarity to your constants, and when used correctly, they can make your codebase more robust and easier to understand.
Ready to level up your TypeScript game? Check out the official TypeScript documentation for more advanced enum features and patterns.
Remember, great code isn’t just about making it work – it’s about making it work elegantly. Happy coding! 🚀
Have questions or want to share your enum experiences? Drop a comment below !
Next: gRPC vs REST: The #1 Thing You Need to Know for Lightning-Fast APIs ⚡