Introduction
Ever felt like your serverless applications are running into performance bottlenecks? Welcome to the world of AWS Lambda concurrency โ your secret weapon for building highly scalable and responsive cloud applications!
What is AWS Lambda Concurrency? ๐ค
Lambda concurrency is like having multiple chefs in a kitchen, simultaneously preparing different dishes without getting in each other’s way. In technical terms, it’s the number of function instances that can process events simultaneously in your AWS Lambda function.
Understanding Lambda Concurrency Fundamentals
Types of AWS Lambda Concurrency
- Reserved Concurrency ๐
- Dedicated capacity for critical functions
- Guarantees a specific number of concurrent executions
- Prevents your most important functions from being throttled
- Provisioned Concurrency ๐๏ธ
- Pre-initialized function instances
- Eliminates cold starts
- Provides predictable performance for latency-sensitive applications
Real-World Concurrency Scenarios
Scenario 1: E-commerce Order Processing ๐
Imagine Black Friday sales where thousands of orders flood your system simultaneously. Lambda concurrency ensures each order gets processed without overwhelming your infrastructure.
import boto3
def process_order(event, context):
# Concurrent order processing logic
order_id = event['order_id']
# Process payment, update inventory
return {
'statusCode': 200,
'body': f'Order {order_id} processed successfully'
}
Scenario 2: Image Processing Pipeline ๐ธ
Upload multiple images, and Lambda handles them concurrently:
def resize_image(event, context):
# Parallel image resizing
s3_client = boto3.client('s3')
# Resize and store images
return {
'statusCode': 200,
'processedImages': len(event['images'])
}
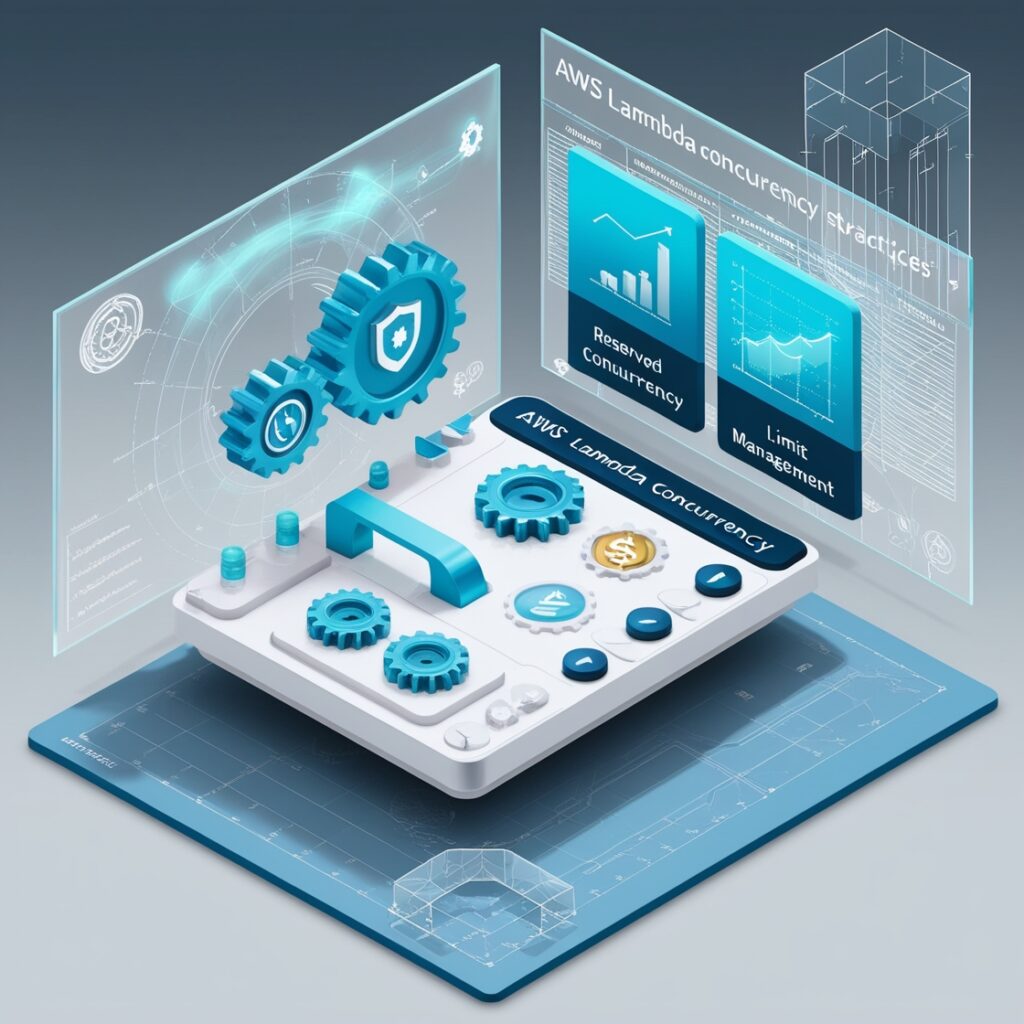
Best Practices for AWS Lambda Concurrency
๐ Pro Tips
- Set Appropriate Limits
- Monitor your AWS account’s default concurrency limit
- Request limit increases strategically
- Use Reserved Concurrency Wisely
- Protect critical functions
- Prevent potential DoS scenarios
- Implement Intelligent Retry Mechanisms
def resilient_function(event, context):
try:
# Primary logic
return process_data(event)
except Exception as e:
# Smart retry logic
retry_count = event.get('retry_count', 0)
if retry_count < 3:
# Retry with exponential backoff
event['retry_count'] = retry_count + 1
return retry_processing(event)
Performance Optimization Strategies ๐
Concurrency Control Techniques
- Circuit Breakers: Prevent system overload
- Rate Limiting: Control request frequency
- Asynchronous Processing: Decouple time-consuming tasks
Cost Implications ๐ฐ
- Concurrency directly impacts your AWS bill
- Optimize by:
- Right-sizing function memory
- Implementing efficient code
- Using provisioned concurrency judiciously
Common Challenges and Solutions ๐ ๏ธ
Challenge: Cold Starts
Solution:
- Use Provisioned Concurrency
- Minimize function initialization time
- Choose lightweight runtimes
Monitoring Concurrency
def lambda_handler(event, context):
# Log concurrency metrics
print(f"Remaining Concurrency: {context.get_remaining_concurrency()}")
FAQ Section โ
Q1: What’s the default AWS Lambda concurrency limit?
A: By default, AWS provides 1,000 concurrent executions per account per region.
Q2: How do I monitor Lambda concurrency?
A: Use CloudWatch metrics, tracking ConcurrentExecutions
and UnreservedConcurrentExecutions
.
Q3: Can I increase my concurrency limit?
A: Yes! Contact AWS support to request a limit increase.
Q4: What happens when I exceed concurrency limits?
A: Incoming requests are throttled, returning a 429 (Too Many Requests) error.
Q5: Is Provisioned Concurrency worth the extra cost?
A: For latency-critical applications, absolutely! It eliminates cold starts and provides predictable performance.
Conclusion ๐
Lambda concurrency isn’t just a technical feature โ it’s your pathway to building responsive, scalable serverless applications. By understanding and implementing smart concurrency strategies, you’ll transform your cloud architecture from good to extraordinary!
Ready to Master Lambda Concurrency? Start Experimenting Today! ๐ก
Disclaimer: Always test and benchmark your specific use cases.
Next: Secure Your App Credentials with AWS Secrets Manager ๐
3 thoughts on “Unlock the Power of AWS Lambda Concurrency for Effortless, Scalable Success๐”